43 indexing using labels in dataframe
How to drop rows in Pandas DataFrame by index labels? Rows can be removed using index label or column name using this method. Syntax: DataFrame.drop (labels=None, axis=0, index=None, columns=None, level=None, inplace=False, errors='raise') Parameters: labels: String or list of strings referring row or column name. axis: int or string value, 0 'index' for Rows and 1 'columns' for Columns. Indexing and selecting data — pandas 1.5.1 documentation Indexing and selecting data# The axis labeling information in pandas objects serves many purposes: Identifies data (i.e. provides metadata) using known indicators, important for analysis, visualization, and interactive console display. Enables automatic and explicit data alignment. Allows intuitive getting and setting of subsets of the data set.
Pandas DataFrame Indexing Streamlined - Table of Contents part of Course 131 Data Munging Tips and Tricks Indexing a Pandas DataFrame for people who don't like to remember things Use loc[] to choose rows and columns by label. Use iloc[] to choose rows and columns by position. Be explicit about both rows and columns, even if it's with ":"
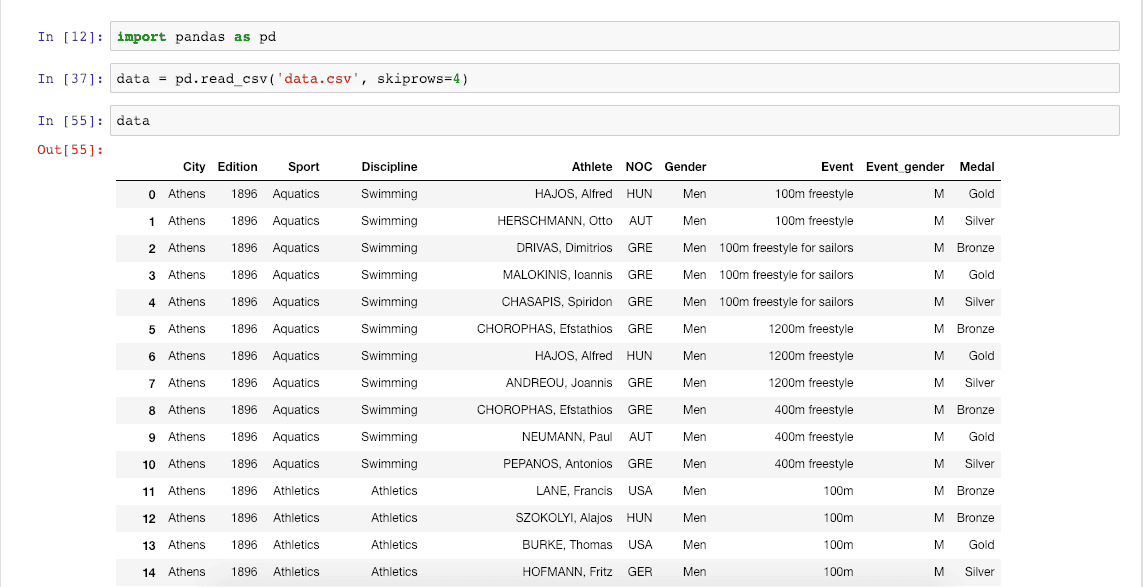
Indexing using labels in dataframe
Intro to data structures — pandas 1.5.1 documentation DataFrame.from_dict. DataFrame.from_dict() takes a dict of dicts or a dict of array-like sequences and returns a DataFrame. It operates like the DataFrame constructor except for the orient parameter which is 'columns' by default, but which can be set to 'index' in order to use the dict keys as row labels. pandas.DataFrame.set_index — pandas 1.5.1 documentation Set the DataFrame index (row labels) using one or more existing columns or arrays (of the correct length). The index can replace the existing index or expand on it. Parameters. keyslabel or array-like or list of labels/arrays. This parameter can be either a single column key, a single array of the same length as the calling DataFrame, or a list ... Get Rows by their Index and Labels - Data Science Parichay Example 1: Select Rows Based on their Integer Indices. Rows and columns are indexed starting from 0 (by default) in a pandas dataframe. As mentioned above, we can use the iloc property to access rows using their integer indices. Let's select the row with the index 2 (the 3rd row) in the dataframe. df.iloc[2]
Indexing using labels in dataframe. 10 minutes to pandas — pandas 1.5.1 documentation DataFrame.to_numpy() gives a NumPy representation of the underlying data. Note that this can be an expensive operation when your DataFrame has columns with different data types, which comes down to a fundamental difference between pandas and NumPy: NumPy arrays have one dtype for the entire array, while pandas DataFrames have one dtype per column. Pandas Dataframe Index in Python - PythonForBeginners.com When a dataframe is created, the rows of the dataframe are assigned indices starting from 0 till the number of rows minus one. However, we can create a custom index for a dataframe using the index attribute. To create a custom index in a pandas dataframe, we will assign a list of index labels to the index attribute of the dataframe. Pandas DataFrame Indexing Explained: from .loc to .iloc and beyond Indexing can be described as selecting values from specific rows and columns in a dataframe. The row labels (the dataframe index) can be integer or string values, the column labels are usually strings. By indexing, we can be very particular about the selections we make, zooming in on the exact data that we need. pandas.DataFrame.loc — pandas 1.5.1 documentation pandas.DataFrame.loc# property DataFrame. loc [source] # Access a group of rows and columns by label(s) or a boolean array..loc[] is primarily label based, but may also be used with a boolean array. Allowed inputs are: A single label, e.g. 5 or 'a', (note that 5 is interpreted as a label of the index, and never as an integer position along the ...
MultiIndex / advanced indexing — pandas 1.5.1 documentation A MultiIndex can be created from a list of arrays (using MultiIndex.from_arrays()), an array of tuples (using MultiIndex.from_tuples()), a crossed set of iterables (using MultiIndex.from_product()), or a DataFrame (using MultiIndex.from_frame()). The Index constructor will attempt to return a MultiIndex when it is passed a list of tuples. The ... Indexing in Pandas Dataframe using Python | by Kaushik Katari | Towards ... Indexing using .loc method. If we use the .loc method, we have to pass the data using its Label name. Single Row To display a single row from the dataframe, we will mention the row's index name in the .loc method. The whole row information will display like this, Single Row information Multiple Rows To get subset of dataframe based on index of a label Question: I have a dataframe from yahoo finance This gives me df as, If I specify, I need a subset of df with row having indexes label "2021-04-23" rows of 2 days before the date row of 1 day after of date The important thing here is, we cannot calculate before & after using date strings as df may not have some dates but rows to be printed based on indexes. Python Pandas: Get Index Label for a Value in a DataFrame I typically do the following using np.where: import numpy as np idx = df.index [np.where (df ['hair'] == 'blonde')] Which gives the expected result: Index ( [u'mary'], dtype='object') If you want the result in a list, you can use .tolist () method of index Share Follow answered Jun 14, 2017 at 15:28 FLab 6,816 3 34 63 Add a comment Your Answer
Tutorial: How to Index DataFrames in Pandas - Dataquest Label-based Dataframe Indexing As its name suggests, this approach implies selecting dataframe subsets based on the row and column labels. Let's explore four methods of label-based dataframe indexing: using the indexing operator [], attribute operator ., loc indexer, and at indexer. Using the Indexing Operator Get Row Labels of a Pandas DataFrame. - Data Science Parichay Dataframe row labels can be obtained using Pandas' dataframe in-built index property. df.index returns a RangeIndex object or an Index object, depending on whether the dataframe df has a default index or a custom index. The underlying row labels can be obtained using df.index.values. The syntax will be-. row_labels = df.index.values. MultiIndex / advanced indexing — pandas 1.5.1 documentation A MultiIndex can be created from a list of arrays (using MultiIndex.from_arrays()), an array of tuples (using MultiIndex.from_tuples()), a crossed set of iterables (using MultiIndex.from_product()), or a DataFrame (using MultiIndex.from_frame()). The Index constructor will attempt to return a MultiIndex when it is passed a list of tuples. The ... The Pandas DataFrame: Make Working With Data Delightful This Pandas DataFrame looks just like the candidate table above and has the following features: Row labels from 101 to 107; Column labels such as 'name', 'city', 'age', and 'py-score' Data such as candidate names, cities, ages, and Python test scores; This figure shows the labels and data from df:
Indexing Dataframes. Indexing Dataframes in Pandas | by Vidya Menon ... A callable function with one argument (the calling Series or DataFrame) and that returns valid output for indexing (one of the above) Using Single label: Helps to specify which rows and/or columns we want .For rows, the label is the index value of that row, and for columns, the column name is the label.
Pandas DataFrame Indexing: Set the Index of a Pandas Dataframe Python list as the index of the DataFrame In this method, we can set the index of the Pandas DataFrame object using the pd.Index (), range (), and set_index () function. First, we will create a Python sequence of numbers using the range () function then pass it to the pd.Index () function which returns the DataFrame index object.
Label-based indexing to the Pandas DataFrame - GeeksforGeeks Indexing plays an important role in data frames. Sometimes we need to give a label-based "fancy indexing" to the Pandas Data frame. For this, we have a function in pandas known as pandas.DataFrame.lookup (). The concept of Fancy Indexing is simple which means, we have to pass an array of indices to access multiple array elements at once.
Get Rows by their Index and Labels - Data Science Parichay Example 1: Select Rows Based on their Integer Indices. Rows and columns are indexed starting from 0 (by default) in a pandas dataframe. As mentioned above, we can use the iloc property to access rows using their integer indices. Let's select the row with the index 2 (the 3rd row) in the dataframe. df.iloc[2]
pandas.DataFrame.set_index — pandas 1.5.1 documentation Set the DataFrame index (row labels) using one or more existing columns or arrays (of the correct length). The index can replace the existing index or expand on it. Parameters. keyslabel or array-like or list of labels/arrays. This parameter can be either a single column key, a single array of the same length as the calling DataFrame, or a list ...
Intro to data structures — pandas 1.5.1 documentation DataFrame.from_dict. DataFrame.from_dict() takes a dict of dicts or a dict of array-like sequences and returns a DataFrame. It operates like the DataFrame constructor except for the orient parameter which is 'columns' by default, but which can be set to 'index' in order to use the dict keys as row labels.
Post a Comment for "43 indexing using labels in dataframe"